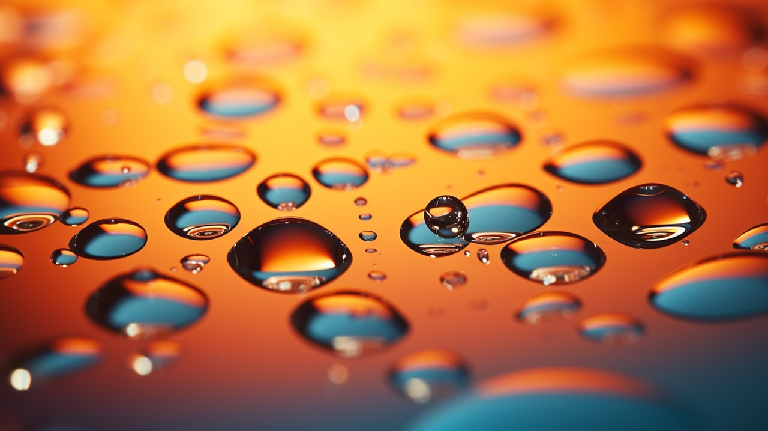
前言
嗨,大家好!
委托是一种强大的工具,可以让你将方法作为参数传递。
在 C# 中,创建委托有多种方法,每种方法都有其特点和适用场景。
我总结了 7 个创建委托的方法,看看有没有你不知道的方法?
1. 使用 delegate 关键字
这是最基本的创建委托的方法,通过 delegate
关键字定义一个委托类型
using System;
// 定义一个委托类型
public delegate void MyDelegate();
class Program
{
static void Main()
{
// 创建委托实例
MyDelegate myDelegate = new MyDelegate(Method1);
// 添加另一个方法到委托
myDelegate += Method2;
// 调用委托
myDelegate();
}
static void Method1()
{
Console.WriteLine("Method1 called");
}
static void Method2()
{
Console.WriteLine("Method2 called");
}
}
2. 使用 Func 泛型委托
C# 提供了内置的泛型委托 Func
委托,它可以简化创建能够返回值的方法委托
using System;
class Program
{
static void Main()
{
// 定义一个 Func 委托,接受两个 int 参数,返回一个 int
Func<int, int, int> add = Add;
// 调用委托
int result = add(10, 5);
Console.WriteLine(result); // 输出: 15
}
static int Add(int a, int b)
{
return a + b;
}
}
Func
委托可以接受最多16个参数,最后一个类型参数是方法的返回值。
3. 使用 Action 泛型委托
C# 提供了内置的泛型委托 Action
委托,它可以简化创建不返回任何值的方法委托
using System;
class Program
{
static void Main()
{
// 定义一个 Action 委托,接受一个 string 参数
Action<string> greet = Greet;
// 调用委托
greet("World"); // 输出: Hello, World
}
static void Greet(string name)
{
Console.WriteLine($"Hello, {name}");
}
}
和 Func
委托一样,Action
委托也可以接受最多16个参数
4. 使用匿名方法
匿名方法允许你在定义委托时直接编写方法体,而不需要单独定义一个方法。
示例
using System;
class Program
{
static void Main()
{
// 定义一个委托类型
delegate void MyDelegate();
// 使用匿名方法创建委托实例
MyDelegate myDelegate = delegate
{
Console.WriteLine("Anonymous method called");
};
// 调用委托
myDelegate();
}
}
5. 使用 Lambda 表达式
Lambda 表达式是匿名方法的简化形式,语法更简洁,使用更方便。
示例
using System;
class Program
{
static void Main()
{
// 定义一个 Action 委托,接受一个 string 参数
Action<string> greet = name => Console.WriteLine($"Hello, {name}");
// 调用委托
greet("World"); // 输出: Hello, World
}
}
6. 使用方法组转换
直接将一个符合委托签名的方法名赋值给委托变量,不需要显式地使用 new
关键字
public delegate void MyDelegate(string message);
public class Program
{
public static void Main()
{
// 直接将方法名赋值给委托
MyDelegate del = PrintMessage;
del("你好,通过方法组转换创建的委托!");
}
public static void PrintMessage(string message)
{
Console.WriteLine(message);
}
}
7. 多播委托
多播委托(multicast delegates)是一种特殊的委托类型,允许一个委托引用多个方法,在调用委托时,依次执行所有引用的方法。
在创建多播委托时,通过 +=
操作符将多个方法添加到一个委托中。
public delegate void MyDelegate(string message);
public class Program
{
public static void Main()
{
MyDelegate del = PrintMessage;
del += AnotherMessage; // 添加第二个方法
del("你好,多播委托!"); // 调用所有注册的方法
}
public static void PrintMessage(string message)
{
Console.WriteLine("PrintMessage: " + message);
}
public static void AnotherMessage(string message)
{
Console.WriteLine("AnotherMessage: " + message);
}
}
需要注意的是,如果多播委托有返回值,那么返回值将是最后一个方法的返回值。在应用中,建议将多播委托用于void类型的返回值的场景,以避免返回最后一个方法的结果。
总结
通过上述几种方法,你可以灵活地创建和使用委托。
delegate
关键字:适用于需要自定义委托类型的情况。Func
和 Action
泛型委托:适用于标准的委托需求,语法简洁。Lambda
表达式:语法简洁,适用于简单的委托。- 多播委托:适用于需要依次调用多个方法的情况,比如在 WinForms 中单击按钮处理多个任务。
该文章在 2024/11/22 16:01:29 编辑过